Starting My Rust Journey
With a few things coming together, I have finally decided to look into yet another programming language. On the one hand learning a new language is usually a good way to broaden the fundamenetal understanding of programming, but this time I do have a specific goal in mind that I want to reach.
Only recently I have been looking at various 32 bit Cortex-M ARM
micro-controllers with the vendor provided C
programming
environments. Knowing modern programming languages like Haskell,
OCaml or Go got me so frustrated with these environments that I
decided to find a modern language also fit for this embedded space and
no, I don't think Python is the answer to every problem just as Java
wasn't. Ultimately, I would like to see a functional programming
language in this space, but I am open to other approaches fitting the
embedded space.
Being an FSF Emacs user for decades, I am also averse to these proprietary programming environments that may have some nice features but ultimately only try to lock the developer into a vendor controlled ecosystem. Usually they are all Eclipse based anyway but are incompatible with one another. It gives me the shivers installing the n-th copy of Eclipse for yet another Cortex-M based micro-controller and I really wonder how we ended up in this very frustrating assembly of walled in environments.
So this is the start of a small series of posts that evaluate how easy it will be to setup a programming environment on GNU/Linux for Rust based on GNU Emacs. Ultimately I want to target micro-controllers without a full GNU/Linux system running on them, so the aim is to also get a cross-compilation setup working.
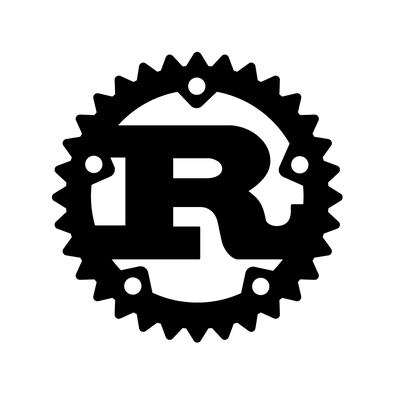
As the The Rust Programming Language book is available online, it doesn't really make sense for me to do a real Rust introduction in this mini-series and so I will try to concentrate on the aspects that go beyond this book. For myself I decided to not only read the book on-line, but also buy the book at a local book store and thus have the best of both world. I still enjoy reading a book, but for the examples it is nice to have the text available in a window next to the IDE. In case you think alike but don't have a nice local book store that you want to support, then you may want to consider ordering it through Bookzilla where 5% of the price will go the Free Software Foundation Europe rather than buying it through Amazon which does not even pay taxes let alone support any causes beyond pure profit.
Installing the base tool chain by default is done in a per-user fashion so every user has full control over all aspects over it. A provided script makes this a no-brainer:
[dzu@harry ~]$ lsb_release -d Description: Ubuntu 18.04.2 LTS [dzu@harry ~]$ curl https://sh.rustup.rs -sSf | sh info: downloading installer Welcome to Rust! This will download and install the official compiler for the Rust programming language, and its package manager, Cargo. It will add the cargo, rustc, rustup and other commands to Cargo's bin directory, located at: /home/dzu/.cargo/bin This path will then be added to your PATH environment variable by modifying the profile file located at: /home/dzu/.profile You can uninstall at any time with rustup self uninstall and these changes will be reverted. Current installation options: default host triple: x86_64-unknown-linux-gnu default toolchain: stable modify PATH variable: yes 1) Proceed with installation (default) 2) Customize installation 3) Cancel installation > info: syncing channel updates for 'stable-x86_64-unknown-linux-gnu' info: latest update on 2019-01-17, rust version 1.32.0 (9fda7c223 2019-01-16) info: downloading component 'rustc' 79.5 MiB / 79.5 MiB (100 %) 6.4 MiB/s ETA: 0 s info: downloading component 'rust-std' 54.3 MiB / 54.3 MiB (100 %) 6.3 MiB/s ETA: 0 s info: downloading component 'cargo' info: downloading component 'rust-docs' 8.5 MiB / 8.5 MiB (100 %) 6.5 MiB/s ETA: 0 s info: installing component 'rustc' info: installing component 'rust-std' info: installing component 'cargo' info: installing component 'rust-docs' info: default toolchain set to 'stable' stable installed - rustc 1.32.0 (9fda7c223 2019-01-16) Rust is installed now. Great! To get started you need Cargo's bin directory ($HOME/.cargo/bin) in your PATH environment variable. Next time you log in this will be done automatically. To configure your current shell run source $HOME/.cargo/env [dzu@harry ~]$
As described, this installs the tool-chain below $HOME/.cargo/bin
and
modifies your $HOME/.profile
to source $HOME/.cargo/env
to set
this up in interactive shells. So on a subsequent session, you do not
have to do anything, but for the current shell we run this only once
to be able to continue:
This gives us easy access to the following command-line tools which we will encounter individually some time further into our journey:
[dzu@harry ~]$ ls ~/.cargo/bin cargo cargo-clippy cargo-fmt rls rustc rustdoc rustfmt rust-gdb rust-lldb rustup [dzu@harry ~]$
One of the drawbacks of such a local install like what we just did is
that there is no documentation that our man
command (and thus
apropos
) will find:
[dzu@harry ~]$ man cargo No manual entry for cargo See 'man 7 undocumented' for help when manual pages are not available. [dzu@harry ~]$
There is however the nice rustup
tool that we will use shortly to
update our installation. Beyond that, it also includes ways to get to
more detailed documentation. Viewing the man page for cargo
can
thus be done with:
You can easily see the list of all available man pages:
[dzu@harry ~]$ ls /home/dzu/.rustup/toolchains/stable-x86_64-unknown-linux-gnu/share/man/man1/ cargo.1 cargo-fetch.1 cargo-new.1 cargo-rustc.1 cargo-version.1 cargo-bench.1 cargo-generate-lockfile.1 cargo-owner.1 cargo-rustdoc.1 cargo-yank.1 cargo-build.1 cargo-init.1 cargo-package.1 cargo-search.1 rustc.1 cargo-check.1 cargo-install.1 cargo-pkgid.1 cargo-test.1 rustdoc.1 cargo-clean.1 cargo-login.1 cargo-publish.1 cargo-uninstall.1 cargo-doc.1 cargo-metadata.1 cargo-run.1 cargo-update.1 [dzu@harry ~]$
There is even a whole copy of the Rust Programming Language book installed locally. Accessing it in your browser is simple:
Before moving on, we ensure that the script really installed the
latest and greatest rust tool-chain by using the rustup
:
[dzu@harry ~]$ rustup update info: syncing channel updates for 'stable-x86_64-unknown-linux-gnu' info: checking for self-updates stable-x86_64-unknown-linux-gnu unchanged - rustc 1.32.0 (9fda7c223 2019-01-16) [dzu@harry ~]$
Ok, so now that we now that we are playing with the latest and
greatest Rust, let's create our first little project. Note that I'm
immediately jumping ahead by using cargo
. After all, one of the
reasons I wanted to look into Rust is to get away from the makefile
madness of C:
[dzu@harry rust]$ cargo new hello_cargo Created binary (application) `hello_cargo` package [dzu@harry rust]$
As simple as that. Let's take a look at what we got:
[dzu@harry rust]$ ls -lR hello_cargo/ hello_cargo/: total 8 -rw-rw-r-- 1 dzu dzu 134 Feb 24 21:27 Cargo.toml drwxrwxr-x 2 dzu dzu 4096 Feb 24 21:27 src hello_cargo/src: total 4 -rw-rw-r-- 1 dzu dzu 45 Feb 24 21:27 main.rs [dzu@harry rust]$ cd hello_cargo/ [dzu@harry hello_cargo (master)]$
As you can see by my git aware PS1 prompt, cargo
even initialized
a git repository for us!
Having checked the contents of the source, let's run our first example right away:
[dzu@harry hello_cargo (master)]$ cat src/main.rs fn main() { println!("Hello, world!"); } [dzu@harry hello_cargo (master)]$ cargo run Compiling hello_cargo v0.1.0 (/home/dzu/src/rust/hello_cargo) Finished dev [unoptimized + debuginfo] target(s) in 0.38s Running `target/debug/hello_cargo` Hello, world! [dzu@harry hello_cargo (master)]$
I sure am impressed with the smoothness of this initial contact. Let's see how easy it is to setup Emacs as an IDE on top of this command line machinery in the next part.
Comments
Comments powered by Disqus